Creating complex file structures is a time-consuming and error-prone task. Let's compare different approaches to create a typical office project structure.
The Target Structure
Here's what we want to achieve — a standard business project structure:
Project2024 ├── Documents │ ├── Contracts │ │ └── PRJ_2024_001_ServiceAgreement.docx │ └── Reports │ ├── Q1 │ │ └── PRJ_2024_Q1_ProgressReport.docx │ └── Q2 │ └── PRJ_2024_Q2_ProgressReport.docx ├── Media │ └── Photos │ └── PRJ_2024_TeamMeeting_Jan.jpg ├── Communications │ ├── Emails │ │ └── PRJ_2024_ClientCorrespondence.pdf │ └── Meetings │ └── PRJ_2024_KickoffMeeting_Minutes.docx └── Resources └── PRJ_2024_ProjectTimeline.xlsx
Let's explore three different ways to create this structure:
1. Creating the structure by hand
This will work, but you won't feel great about it. Your time should be spent better than that. For very simple structures, this is fine. But for more complex operations, you'll want to use a more powerful tool.
2. Bash Scripting
Shell script are powerful. They can be used to create file structures. They can be a bit hard to debug, and require some time to learn, but they can be a good option.
1#!/bin/bash
2
3# Define array of files and directories
4files=(
5 "Documents/Contracts/PRJ_2024_001_ServiceAgreement.docx"
6 "Documents/Reports/Q1/PRJ_2024_Q1_ProgressReport.docx"
7 "Documents/Reports/Q2/PRJ_2024_Q2_ProgressReport.docx"
8 "Media/Photos/PRJ_2024_TeamMeeting_Jan.jpg"
9 "Communications/Emails/PRJ_2024_ClientCorrespondence.pdf"
10 "Communications/Meetings/PRJ_2024_KickoffMeeting_Minutes.docx"
11 "Resources/PRJ_2024_ProjectTimeline.xlsx"
12)
13
14# Create base project directory
15mkdir -p Project2024
16
17# Create files and their parent directories
18for file in "${files[@]}"; do
19 mkdir -p "Project2024/$(dirname "$file")"
20 touch "Project2024/$file"
21done
3. Python Approach
Python can also be good at this.
1from pathlib import Path
2
3def create_files_and_folders(base_dir: str, structure: dict) -> None:
4 base = Path(base_dir)
5
6 for folder, files in structure.items():
7 folder_path = base / folder
8 folder_path.mkdir(parents=True, exist_ok=True)
9 for file in files:
10 (folder_path / file).touch()
11
12if __name__ == "__main__":
13 structure = {
14 "Documents/Contracts": [
15 "PRJ_2024_001_ServiceAgreement.docx"
16 ],
17 "Documents/Reports/Q1": [
18 "PRJ_2024_Q1_ProgressReport.docx"
19 ],
20 "Documents/Reports/Q2": [
21 "PRJ_2024_Q2_ProgressReport.docx"
22 ],
23 "Media/Photos": [
24 "PRJ_2024_TeamMeeting_Jan.jpg"
25 ],
26 "Communications/Emails": [
27 "PRJ_2024_ClientCorrespondence.pdf"
28 ],
29 "Communications/Meetings": [
30 "PRJ_2024_KickoffMeeting_Minutes.docx"
31 ],
32 "Resources": [
33 "PRJ_2024_ProjectTimeline.xlsx"
34 ]
35 }
36 create_files_and_folders("Project2024", structure)
4. File Architect
If you don't really want to write code, or just want to use a tool that is more visually representative of the structure you want to create, you can use the File Architect. It's a simple app that allows you to generate the structure from a readable text format. Hierarchy is defined by indentation and no coding knowledge is not required. This will also allow you to easily copy files and folder structures from elsewhere, use file replacements, create templates, and more.
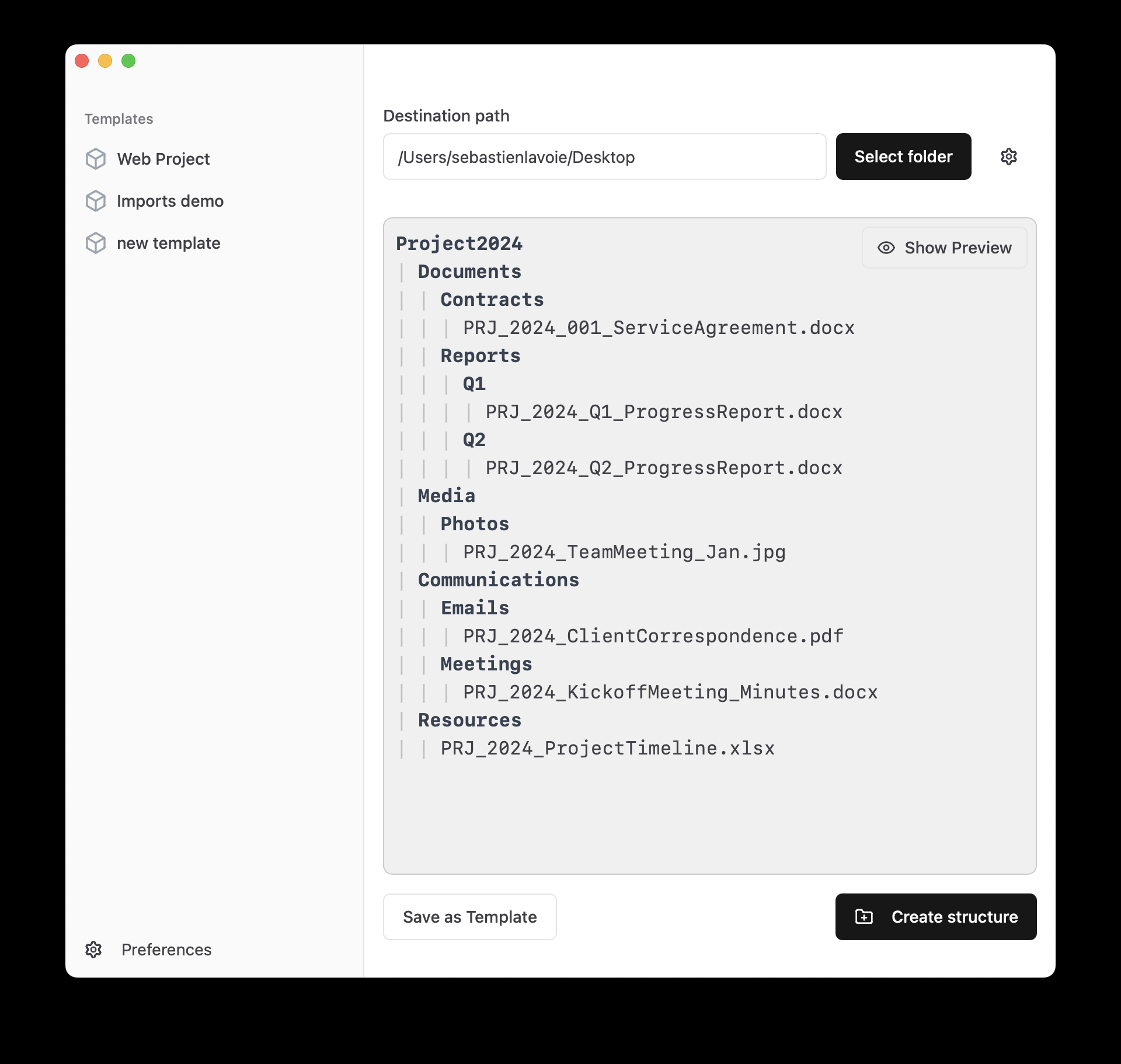
Project2024 Documents Contracts PRJ_2024_001_ServiceAgreement.docx Reports Q1 PRJ_2024_Q1_ProgressReport.docx Q2 PRJ_2024_Q2_ProgressReport.docx Media Photos PRJ_2024_TeamMeeting_Jan.jpg Communications Emails PRJ_2024_ClientCorrespondence.pdf Meetings PRJ_2024_KickoffMeeting_Minutes.docx Resources PRJ_2024_ProjectTimeline.xlsx
Conclusion:
While both Bash and Python offer good solutions to create basic file structures, File Architect provides a more intuitive, more powerful, visual approach that's easier to use for non-coders.
Is this article biased? Of course it is. But I sincerly built this tool for myself as I thought those coding approaches were too tedious. So give it a try,you can use it for free for 7 days! Get Started